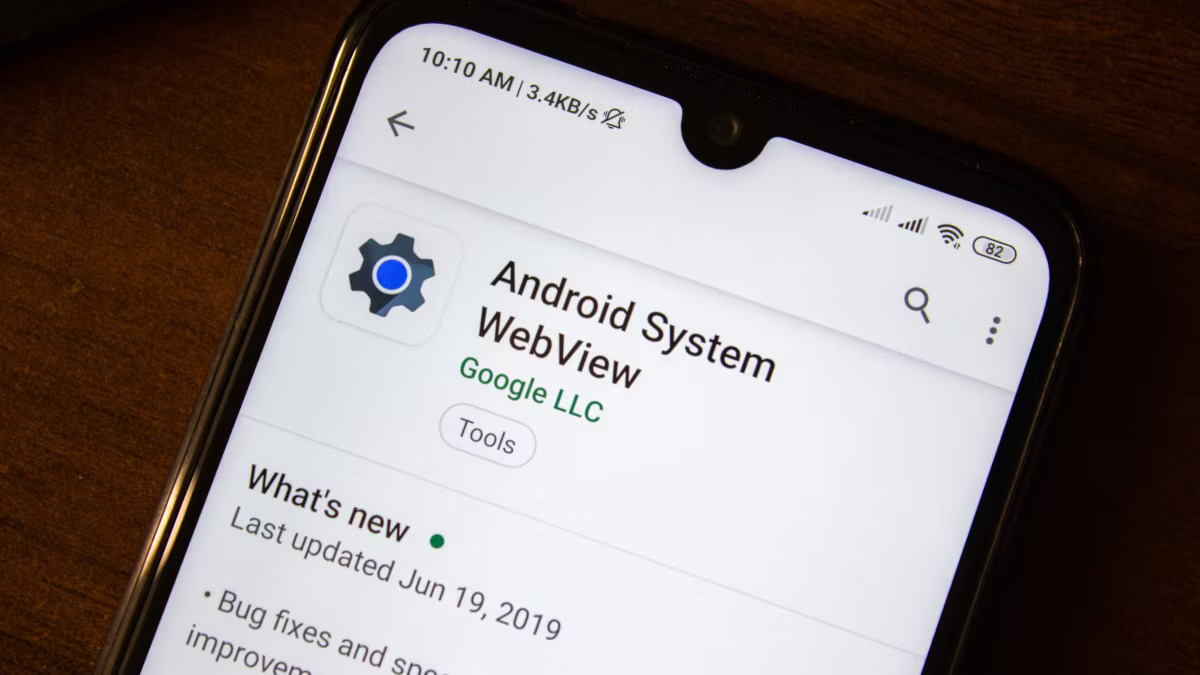
Introduction
Android WebView is a component of the Android operating system that allows developers to embed web pages directly into their applications. It provides a way to display web content within an app, enabling users to interact with web-based services without leaving the app. This article explores Android WebView, covering its history, functionality, usage, and best practices.
What is Android System WebView?
Android System WebView is a system component that allows Android apps to display web pages. Essentially, it is a browser engine running within the context of an application, providing seamless integration of web content into native apps. This component is crucial for many applications, including those requiring users to interact with web-based services or display dynamic content.
History of Android WebView
The concept of embedding web pages within native applications dates back to the early days of mobile development. However, Android's implementation of WebView has evolved significantly over the years. Here’s a brief overview of its history:
- Early Days: The first versions of Android introduced basic support for web views, allowing developers to embed simple web pages into their applications.
- Android 2.x: WebView started to gain more prominence, enabling more complex web-based interfaces within apps.
- Android 3.x: Honeycomb (Android 3.x) made WebView more robust and deeply integrated into the operating system.
- Android 4.x: Jelly Bean brought significant improvements to WebView, including better performance and security features.
- Android 5.x: Lollipop introduced Material Design, enhancing the look and feel of web views within apps.
- Android 6.x: Marshmallow added advanced security features like runtime permissions for better user protection.
- Android 7.x: Nougat continued the trend of improving performance and security.
- Android 8.x: Oreo introduced Android Oreo WebView, designed to be more secure and efficient.
- Android 9.x: Pie saw further refinements in performance and security.
- Android 10.x: Updates improved compatibility with modern web standards.
- Android 11.x: New features like improved performance and better support for modern web technologies were introduced.
- Android 12.x: Enhanced performance and security features continued to be a focus.
How Does Android WebView Work?
Android WebView uses the Chromium browser engine under the hood. This engine is responsible for rendering web pages and handling user interactions. Here’s a detailed breakdown of how it functions:
Rendering Web Pages
When an app uses Android WebView to display a web page, the following process occurs:
- Request: The app requests the URL of the web page it wants to display.
- Loading: The requested URL is sent to the Chromium browser engine, which begins loading the page.
- Rendering: Once the page is loaded, the engine renders it into a visual format that can be displayed on the screen.
- Displaying: The rendered page is then displayed within the app’s interface.
Handling User Interactions
Android WebView not only displays web pages but also handles user interactions such as clicking links, submitting forms, and navigating through pages:
- Event Handling: When a user interacts with a web page (e.g., clicks a link), the event is captured by the Chromium engine.
- Dispatching Events: These events are then dispatched to the app’s JavaScript interface, allowing it to handle them accordingly.
- App Response: The app can respond to these events by executing JavaScript code or sending requests back to the server.
Security Features
Security is a critical aspect of any component used in mobile applications, especially when dealing with web content:
- Runtime Permissions: Introduced in Marshmallow (Android 6.x), runtime permissions ensure that apps can only access certain features or data after obtaining explicit user consent.
- Data Storage: WebView provides secure storage options for data such as cookies and local storage, ensuring that sensitive information remains protected.
- Content Security Policy (CSP): This feature helps prevent cross-site scripting attacks by defining which sources of content are allowed to be executed within a web page.
Usage of Android WebView
Android WebView is versatile and can be used in various scenarios within an application:
Displaying Static Content
One common use case is displaying static HTML content directly within an app:
- Embedding HTML Files: Developers can embed HTML files directly into their app’s resources folder.
- Loading Local Files: These files can be loaded into WebView using the
loadDataWithBaseURL
method.
Dynamic Content
For dynamic content, developers often use JavaScript to fetch data from servers and update the web page accordingly:
- JavaScript Injection: Developers can inject JavaScript code into the web page using the
evaluateJavascript
method. - Ajax Requests: JavaScript can be used to make AJAX requests to fetch data from servers and update the page dynamically.
Hybrid Apps
Many hybrid apps use WebView as a primary component for displaying web content:
- Cross-Platform Development: Tools like React Native and Flutter often use WebView to provide cross-platform compatibility with web-based technologies.
- Native-Like Experience: By using native components like WebView, hybrid apps can achieve a native-like experience while leveraging web development frameworks.
Best Practices
To get the most out of Android WebView, here are some best practices:
- Optimize Performance: Ensure that your web pages are optimized for mobile devices by using responsive design techniques.
- Use Secure Protocols: Always use secure protocols like HTTPS to protect user data.
- Handle Errors Gracefully: Implement error handling mechanisms to ensure that your app remains stable even when encountering issues with web pages.
- Keep Up-to-Date: Regularly update your app to ensure compatibility with the latest versions of WebView and Android OS.
Common Issues and Solutions
While Android WebView is powerful, it can sometimes encounter issues that need to be addressed:
Crashes and Freezes
If your app crashes or freezes when using WebView, here are some troubleshooting steps:
- Check Logs: Analyze the logs to identify any errors or exceptions related to WebView.
- Update Dependencies: Ensure that all dependencies including Chromium engine are up-to-date.
- Optimize Resources: Optimize resource usage by reducing the complexity of web pages or using more efficient rendering techniques.
Performance Issues
Performance issues can arise due to various reasons such as complex web pages or inefficient rendering:
- Simplify Web Pages: Simplify complex web pages by removing unnecessary elements or optimizing images.
- Use Offline Cache: Use offline cache mechanisms provided by WebView to reduce load times when accessing frequently visited pages.
Final Thoughts
Android WebView is an essential component for any Android application that requires displaying web content. Its evolution over the years has made it more robust and secure, capable of handling complex web-based interfaces seamlessly within native apps. By understanding how it works, its usage scenarios, best practices, and common issues, developers can effectively integrate web content into their Android applications. Whether building hybrid apps or integrating web services into native applications, Android WebView remains an indispensable tool in mobile development.