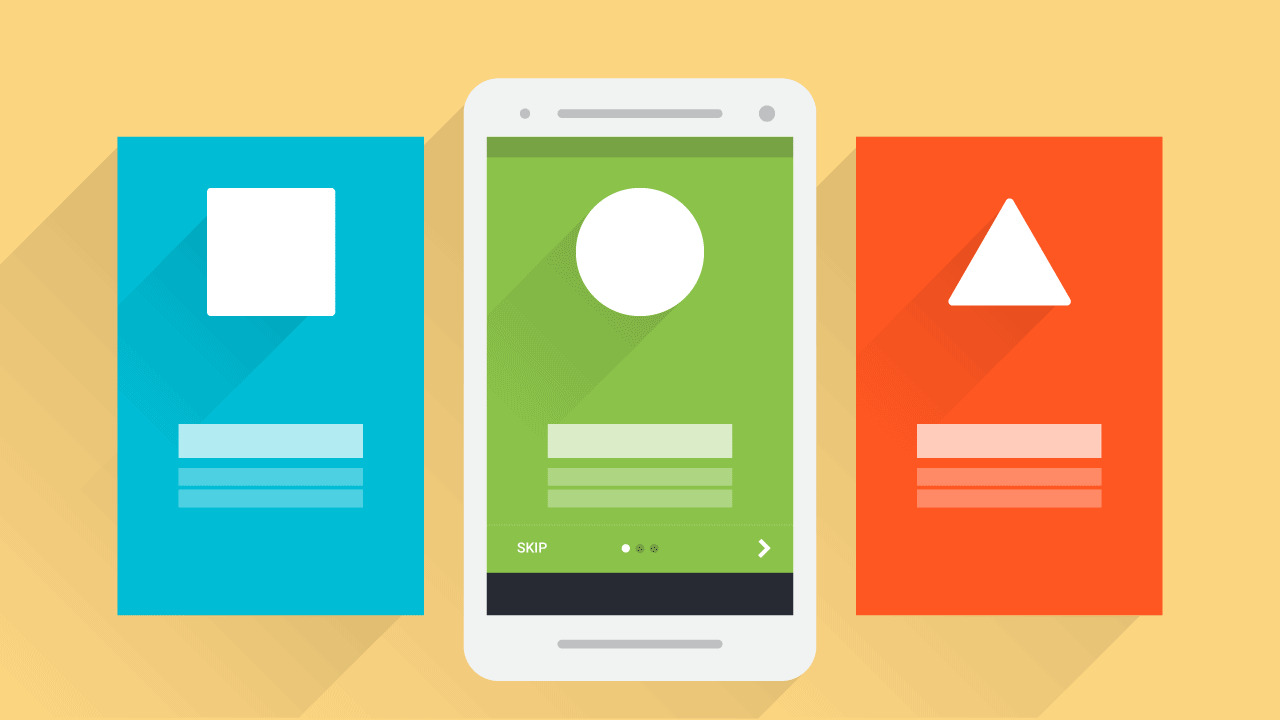
Introduction
Creating an engaging and user-friendly interface is vital in Android app development. One effective way to achieve this is by using the ViewPager
widget. This widget allows users to swipe through pages of data, making it ideal for applications requiring smooth and intuitive navigation. This article will cover ViewPager
features, implementation, and best practices.
Key Takeaways:
- ViewPager2 makes it easy to create swipeable screens in Android apps, like flipping through pages in a book, and it's faster and more flexible than the old ViewPager.
- You can use ViewPager2 to build cool features like image galleries, step-by-step forms, and onboarding screens, making your app more interactive and fun to use!
What is ViewPager?
ViewPager
is a widget in the Android Support Library that enables users to swipe through pages of data. Designed to display a sequence of pages, each can be a fragment or a view. The ViewPager
widget provides a built-in swipe gesture to transition through these pages, making it a versatile tool for various applications.
Why Use ViewPager?
Several reasons make ViewPager
a valuable addition to your Android application:
- User Experience: The swipe gesture provided by
ViewPager
is intuitive and easy to use, enhancing the overall user experience. - Flexibility: Use either views or fragments as the content for each page, giving flexibility in structuring your application.
- Customization: The
PagerAdapter
interface allows customization of the behavior and appearance of the pages, making it easy to tailor theViewPager
to specific needs. - Animation: The
ViewPager
automatically handles animations for screen slides, providing smooth transitions between pages.
Implementing ViewPager
Add Dependencies
Add the following dependency in your build.gradle
file if using the support library:
gradle
implementation 'androidx.viewpager2:viewpager2:1.0.0'
For the older version of the support library, use:
gradle
implementation 'com.android.support:design:28.0.0'
Create Layout
In your layout file, add a ViewPager
widget. For example, in activity_main.xml
, add:
xml
<androidx.viewpager2.widget.ViewPager
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/viewpager"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
Create Adapter
To supply the pages to the ViewPager
, create an adapter. Two types of adapters can be used: PagerAdapter
and FragmentStatePagerAdapter
.
Using PagerAdapter
The PagerAdapter
interface supplies views to the ViewPager
. Here’s an example of creating a custom adapter:
java
public class CustomPagerAdapter extends PagerAdapter {
private Context context;
private List
public CustomPagerAdapter(Context context, List<ModelObject> modelObjects) {
this.context = context;
this.modelObjects = modelObjects;
}
@Override
public int getCount() {
return modelObjects.size();
}
@Override
public boolean isViewFromObject(View view, Object object) {
return view == object;
}
@Override
public Object instantiateItem(ViewGroup container, int position) {
View view = LayoutInflater.from(context).inflate(modelObjects.get(position).getLayoutResId(), container, false);
container.addView(view);
return view;
}
@Override
public void destroyItem(ViewGroup container, int position, Object object) {
container.removeView(object);
}
@Override
public CharSequence getPageTitle(int position) {
return modelObjects.get(position).getTitleResId();
}
}
Using FragmentStatePagerAdapter
The FragmentStatePagerAdapter
is used when fragments serve as the content for each page. Here’s an example of creating a custom adapter using fragments:
java
public class ScreenSlidePagerAdapter extends FragmentStatePagerAdapter {
private static final int NUM_PAGES = 5;
public ScreenSlidePagerAdapter(FragmentManager fm) {
super(fm);
}
@Override
public Fragment getItem(int position) {
return new ScreenSlidePageFragment();
}
@Override
public int getCount() {
return NUM_PAGES;
}
}
Setting Up the ViewPager
Instantiate the ViewPager
in your activity and set the adapter. Here’s an example:
java
public class MainActivity extends AppCompatActivity {
private ViewPager viewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
viewPager = findViewById(R.id.viewpager);
viewPager.setAdapter(new CustomPagerAdapter(this, getModelObjects()));
}
private List<ModelObject> getModelObjects() {
List<ModelObject> modelObjects = new ArrayList<>();
// Add your model objects here
return modelObjects;
}
}
Handling Page Transitions
The ViewPager
automatically handles animations for screen slides, but you can customize the behavior by overriding the onPageSelected
and onPageScrolled
methods in your activity.
java
public class MainActivity extends AppCompatActivity {
private ViewPager viewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
viewPager = findViewById(R.id.viewpager);
viewPager.setAdapter(new CustomPagerAdapter(this, getModelObjects()));
viewPager.addOnPageChangeListener(new ViewPager.OnPageChangeListener() {
@Override
public void onPageScrolled(int position, float positionOffset, int positionOffsetPixels) {
// Handle page scrolled event
}
@Override
public void onPageSelected(int position) {
// Handle page selected event
}
@Override
public void onPageScrollStateChanged(int state) {
// Handle page scroll state changed event
}
});
}
private List<ModelObject> getModelObjects() {
List<ModelObject> modelObjects = new ArrayList<>();
// Add your model objects here
return modelObjects;
}
}
Best Practices
Keep these best practices in mind when using ViewPager
:
- Use Fragments: Using fragments with
FragmentStatePagerAdapter
is recommended for complex layouts and dynamic content. - Customize Adapter: Tailor the adapter to fit specific needs, such as adding custom animations or handling different types of pages.
- Handle Page Transitions: Override the
onPageSelected
andonPageScrolled
methods to manage custom page transitions and events. - Test Thoroughly: Ensure the
ViewPager
works as expected, especially on different screen sizes and orientations.
Final Thoughts
The ViewPager
is a powerful widget in Android that provides a smooth and intuitive way to navigate through pages of data. By understanding how to implement and customize the ViewPager
, you can create engaging and user-friendly applications. Whether creating an intro screen, a welcome walkthrough, or a complex data-driven application, the ViewPager
is an essential tool in your Android development toolkit.
Understanding Android ViewPager
Android ViewPager allows users to swipe left or right to navigate between different pages or screens. It’s like flipping through a book, but on your phone. Key functionalities include smooth transitions, support for both horizontal and vertical swiping, and the ability to load fragments or views dynamically. It also integrates well with TabLayout for easy tab navigation.
Necessary Requirements and Compatibility
To use Android ViewPager, your device must meet specific requirements. First, ensure your device runs Android 4.0 (Ice Cream Sandwich) or later. Older versions won't support this feature. Next, check if your device has at least 1GB of RAM. While ViewPager can run on less, performance may suffer.
Your device should also have a multi-touch screen. ViewPager relies on gestures like swiping, so a single-touch screen won't cut it. Make sure your device's screen resolution is at least 480x800 pixels. Lower resolutions might not display content properly.
For developers, ensure your development environment includes Android Studio 3.0 or later. This version supports the necessary libraries and tools. Also, include the ViewPager2 library in your project. Add this line to your build.gradle
file: implementation 'androidx.viewpager2:viewpager2:1.0.0'
.
Lastly, check if your device has Google Play Services. Some features within ViewPager might require it. If you're using custom ROMs or devices without Google Play, you might face compatibility issues.
In summary, you need Android 4.0+, 1GB RAM, multi-touch screen, 480x800 resolution, Android Studio 3.0+, ViewPager2 library, and Google Play Services for full compatibility.
How to Set Up ViewPager
Open Android Studio: Launch the program on your computer.
Create a New Project: Click on "Start a new Android Studio project."
Select Empty Activity: Choose "Empty Activity" and click "Next."
Name Your Project: Enter a name for your project, then click "Finish."
Add Dependencies: Open
build.gradle
(Module: app) and add: groovy implementation 'androidx.viewpager2:viewpager2:1.0.0' implementation 'com.google.android.material:material:1.3.0'Click "Sync Now."
Create Layout File: In
res/layout
, create a new XML file namedactivity_main.xml
and add: xml <androidx.viewpager2.widget.ViewPager2 android:id="@+id/viewPager" android:layout_width="match_parent" android:layout_height="match_parent" />Create Fragment Layout: In
res/layout
, create another XML file namedfragment_sample.xml
and add: xmlCreate Fragment Class: In
java
directory, create a new Java class namedSampleFragment.java
and add: java public class SampleFragment extends Fragment { @Nullable @Override public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { return inflater.inflate(R.layout.fragment_sample, container, false); } }Set Up Adapter: Create a new Java class named
ViewPagerAdapter.java
and add: java public class ViewPagerAdapter extends FragmentStateAdapter { public ViewPagerAdapter(@NonNull FragmentActivity fragmentActivity) { super(fragmentActivity); } @NonNull @Override public Fragment createFragment(int position) { return new SampleFragment(); } @Override public int getItemCount() { return 3; // Number of pages } }Modify MainActivity: Open
MainActivity.java
and add: java public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); ViewPager2 viewPager = findViewById(R.id.viewPager); viewPager.setAdapter(new ViewPagerAdapter(this)); } }Run Your App: Click the green play button to build and run your app on an emulator or connected device.
Tips for Effective Use
Keep it simple: Use ViewPager for swiping between fragments or pages. Avoid overloading with too many elements.
Optimize performance: Use FragmentStatePagerAdapter for large sets of pages. It saves memory by destroying fragments not in view.
Smooth transitions: Implement PageTransformer for custom animations. It enhances user experience with smooth visual effects.
Lazy loading: Load data only when the page is visible. This reduces initial load time and improves performance.
Indicator: Add a TabLayout or DotsIndicator to show current page. It helps users know their position within the content.
Accessibility: Ensure content descriptions for screen readers. This makes your app usable for everyone.
Testing: Test on various devices and screen sizes. This ensures consistent behavior across different environments.
Memory management: Manage fragment lifecycle properly. Avoid memory leaks by detaching listeners and callbacks.
User feedback: Provide visual feedback on swipe actions. This can be done with subtle animations or color changes.
Documentation: Comment your code and document usage. This helps future developers understand your implementation.
Troubleshooting Common Problems
Screen freezing often happens due to too many apps running. Close unused apps to free up memory. If the problem persists, restart the device.
Battery draining quickly can result from high screen brightness or background apps. Lower brightness and close background apps. Also, check for battery-draining apps in settings.
Slow performance might be caused by low storage. Delete unnecessary files or apps. Clearing cache can also help speed things up.
Wi-Fi not connecting could be due to router issues or incorrect settings. Restart the router and ensure the device is within range. Forget the network and reconnect with the correct password.
Apps crashing frequently may need updates. Check the app store for updates. If updated, try reinstalling the app.
Bluetooth not pairing often requires resetting connections. Turn Bluetooth off and on again. Ensure the device is discoverable and within range.
Overheating can occur from prolonged use or heavy apps. Give the device a break and avoid using it while charging. Remove any case that might trap heat.
Touchscreen unresponsive might need a restart. If restarting doesn’t work, check for software updates. Clean the screen to ensure no dirt is causing issues.
No sound during calls could be due to volume settings. Ensure the volume is up and not on mute. Check if the speaker works with other apps.
Camera not working might need a restart. If restarting doesn’t help, clear the camera app’s cache. Ensure no other apps are using the camera.
Privacy and Security Tips
When using Android ViewPager, user data handling becomes crucial. Always ensure data encryption during transmission and storage. Avoid storing sensitive information directly on the device. Implement permissions carefully, only requesting what’s necessary. Regularly update your app to patch security vulnerabilities. Use secure coding practices to prevent data breaches. For maintaining privacy, educate users on privacy settings and offer clear options to control their data. Encourage using strong passwords and two-factor authentication. Finally, always comply with privacy laws like GDPR or CCPA to protect user information.
Comparing Alternatives
Pros of Android ViewPager:
- Smooth Swiping: Allows users to swipe between different screens smoothly.
- Customizable: Developers can easily customize the appearance and behavior.
- Fragment Support: Works well with fragments, making it easier to manage different sections.
- Adapter Flexibility: Supports various adapters like FragmentPagerAdapter and PagerAdapter.
- TabLayout Integration: Integrates seamlessly with TabLayout for tabbed navigation.
Cons of Android ViewPager:
- Complexity: Can be complex to implement for beginners.
- Performance Issues: May cause performance issues with many fragments.
- Limited Animation: Default animations are limited and may require extra coding for custom animations.
- Memory Usage: High memory usage with many fragments or views.
- Lifecycle Management: Managing fragment lifecycles can be tricky.
Alternatives:
RecyclerView with SnapHelper:
- Pros: More control over item views, better performance with large data sets.
- Cons: Requires more setup and custom code.
ViewPager2:
- Pros: Improved performance, better lifecycle handling, supports RecyclerView.
- Cons: Still relatively new, may have fewer resources and examples.
HorizontalScrollView:
- Pros: Simple to implement, good for static content.
- Cons: Lacks built-in paging and adapter support.
Navigation Component:
- Pros: Simplifies navigation, better handling of back stack.
- Cons: More suited for entire app navigation rather than just swiping between screens.
Understanding Android ViewPager
Android ViewPager is a powerful tool for creating swipeable views in your app. It allows users to navigate between different pages with a simple swipe gesture. This enhances user experience by making navigation smooth and intuitive. Implementing ViewPager involves using a PagerAdapter to manage the pages and a ViewPager widget to display them. You can customize the behavior and appearance to fit your app's needs.
Mastering ViewPager can significantly improve your app's usability. It’s essential to keep your code clean and well-organized to avoid performance issues. Testing on various devices ensures compatibility and a seamless experience for all users.
By integrating ViewPager effectively, you can create dynamic, user-friendly interfaces that keep users engaged. Whether you're building a photo gallery, a tutorial, or any other multi-page feature, ViewPager is a versatile solution that can elevate your app's functionality.
What is the use of ViewPager in Android?
ViewPager in Android lets users flip left and right through pages of data. It’s great for swiping through different views with images and texts.
What's the difference between ViewPager and ViewPager2?
ViewPager2 is an updated version of ViewPager. It’s simpler, more functional, and easier to use than the older ViewPager.
Is ViewPager deprecated?
Yes, ViewPager has been replaced by ViewPager2. You should use ViewPager2 for new projects.
How do you create a full image viewer using ViewPager?
First, create an adapter for ViewPager. Then, reference ViewPager from the XML and set the adapter in the MainActivity.java file. Create an array of integers containing the images to show in ViewPager.
Can ViewPager2 handle vertical scrolling?
Yes, ViewPager2 supports both horizontal and vertical scrolling, unlike the original ViewPager.
How do you migrate from ViewPager to ViewPager2?
Follow the ViewPager2 migration guide provided by Android. It includes steps to replace ViewPager with ViewPager2 in your project.
What are the advantages of using ViewPager2?
ViewPager2 offers better performance, easier integration with RecyclerView, and support for vertical scrolling. It’s also more flexible and easier to use.