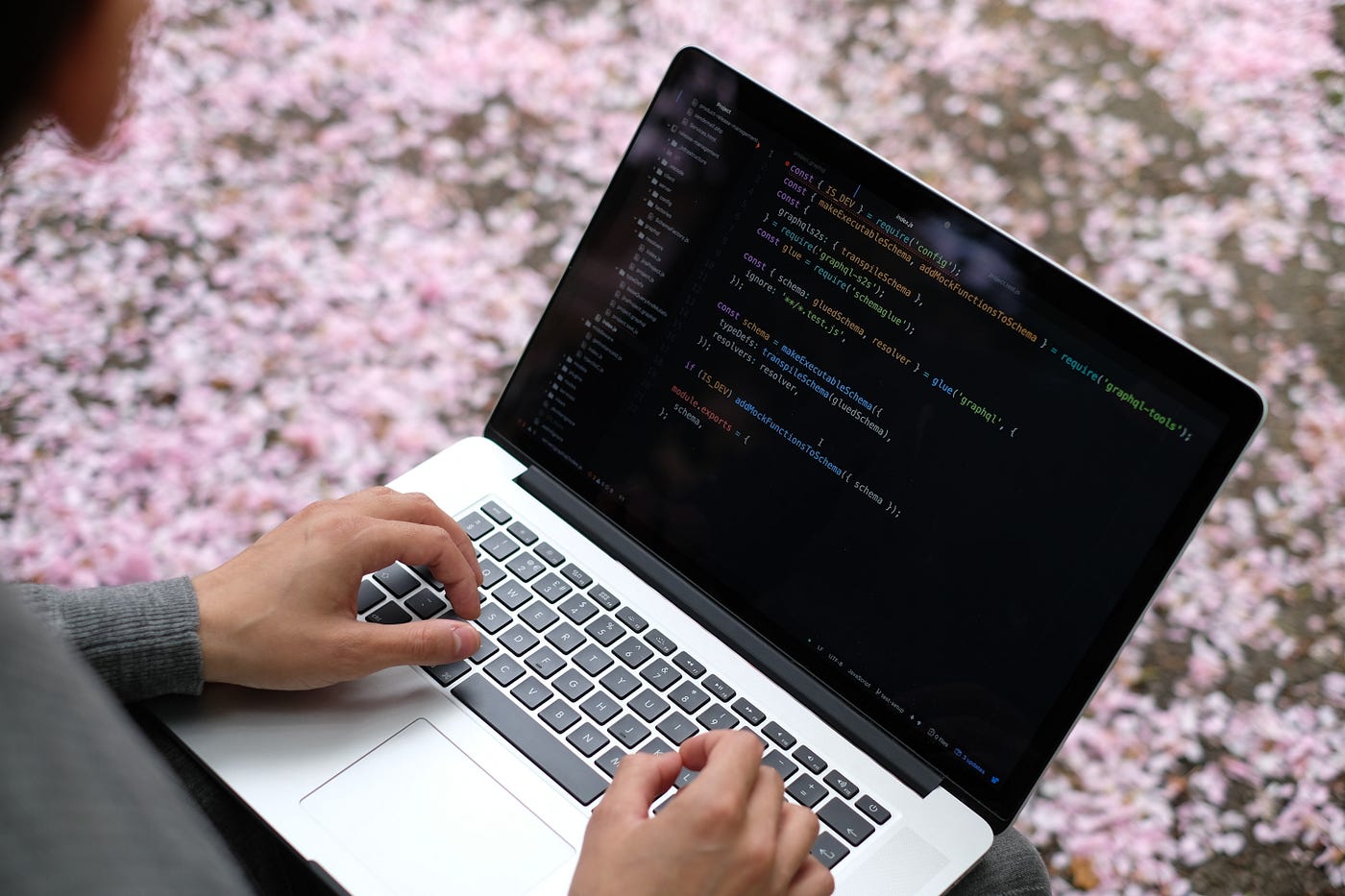
Understanding Reference Issues
Reference issues occur when the compiler cannot locate a specific identifier or class used in the code. These issues can stem from several sources:
- Incorrect Imports: Missing or incorrect import statements prevent the compiler from recognizing referenced classes or methods.
- Missing Dependencies: Dependencies are essential libraries or modules required by your project. If not properly set up, the compiler may fail to find necessary classes.
- Errors in Resource Files: Resource files like layout XML files can contain errors that hinder the generation of the R class, crucial for accessing resources.
- Typo Errors: Simple typos in class names, method calls, or variable declarations can lead to unresolved references.
Resolving Reference Issues: Step-by-Step Guide
Checking Imports
- Identify the Unresolved Reference: Examine the error message to pinpoint the problematic class or method.
- Check for Missing Imports: Review your code for necessary import statements.
- Add Missing Imports: Include any missing import statements.
java
import com.example.yourpackage.YourCustomClass;
Ensuring Dependencies Are Properly Set Up
- Check Gradle File: Open
build.gradle
and verify all necessary dependencies are included. - Add Missing Dependencies: If a dependency is missing, add it to
build.gradle
.
groovy
dependencies {
implementation 'com.example.yourlibrary:yourlibrary:1.0'
}
- Sync Project: Click Sync Now in the toolbar after updating dependencies.
Debugging Resource Files
- Check Layout XML Files: Open layout XML files to find syntax errors or missing attributes.
- Fix Errors: Correct any errors found in the layout XML files.
xml
<Button
android:id="@+id/button"
android:text="Click Me" />
- Clean and Rebuild Project: Click Build > Clean Project followed by Build > Rebuild Project.
Handling Typo Errors
- Review Code: Carefully review your code for typos in class names, method calls, or variable declarations.
- Correct Typos: Fix any typos found in your code.
java
// Corrected code
public void onClick(View view) {
switch (view.getId()) {
case R.id.button:
break;
}
}
Using Quick Fixes in Android Studio
- Invoke Quick Fix Intentions: Android Studio often suggests quick fixes for unresolved references.
- Import R Class: Follow these steps if the IDE suggests importing the R class:
- Place the cursor on the unresolved reference.
- Press
Alt + Enter
(orOption + Enter
on macOS) to invoke quick fix intentions. - Select the option to import the R class from your project.
java
// Corrected code after importing R class
setContentView(R.layout.activity_main);
Analyzing Gradle Build Output
- Check Gradle Build Output: Open the Gradle console by clicking View > Tool Windows > Gradle.
- Look for Errors: Examine the Gradle build output for error messages indicating why the R class is not generated correctly.
- Fix Gradle Errors: Correct any errors found in the Gradle build output.
Invalidating Caches and Restarting
- Invalidate Caches and Restart: Click File > Invalidate Caches / Restart.
- Wait for Restart: Allow Android Studio to restart and invalidate its caches.
Advanced Troubleshooting Techniques
Debugging Dependency Resolution Errors
-
Analyze Dependency Graph: Use the
androidDependencies
task in Gradle to analyze your dependency graph.- Double-click on
AppName
> Tasks > android > androidDependencies. - The Run window will display the output after Gradle executes this task.
- Double-click on
-
Identify Conflicting Dependencies: Look for dependencies appearing more than once or with conflicting versions.
- Select Navigate > Class from the menu bar.
- Ensure the box next to Include non-project items is checked in the pop-up search dialog.
- Type the name of the class appearing in the build error.
- Inspect results for dependencies including this class.
-
Resolve Conflicts: Remove duplicate dependencies or ensure each module uses the same version of a dependency.
Custom Dependency Resolution Strategies
- Custom Build Logic: For guidance on fixing dependency resolution errors involving custom build logic, refer to the Android documentation.
- Inspect Dependency Tree: Identify which dependencies contribute to errors by inspecting your app's dependency tree.
- Remove Duplicate Dependencies: Eliminate duplicate dependencies if they appear more than once in your project.
Resolving reference issues in Android Studio involves understanding their causes and using the right tools and techniques to debug them. By following the steps outlined above, developers can effectively manage reference issues, ensuring smoother development workflows and faster time-to-market for applications.