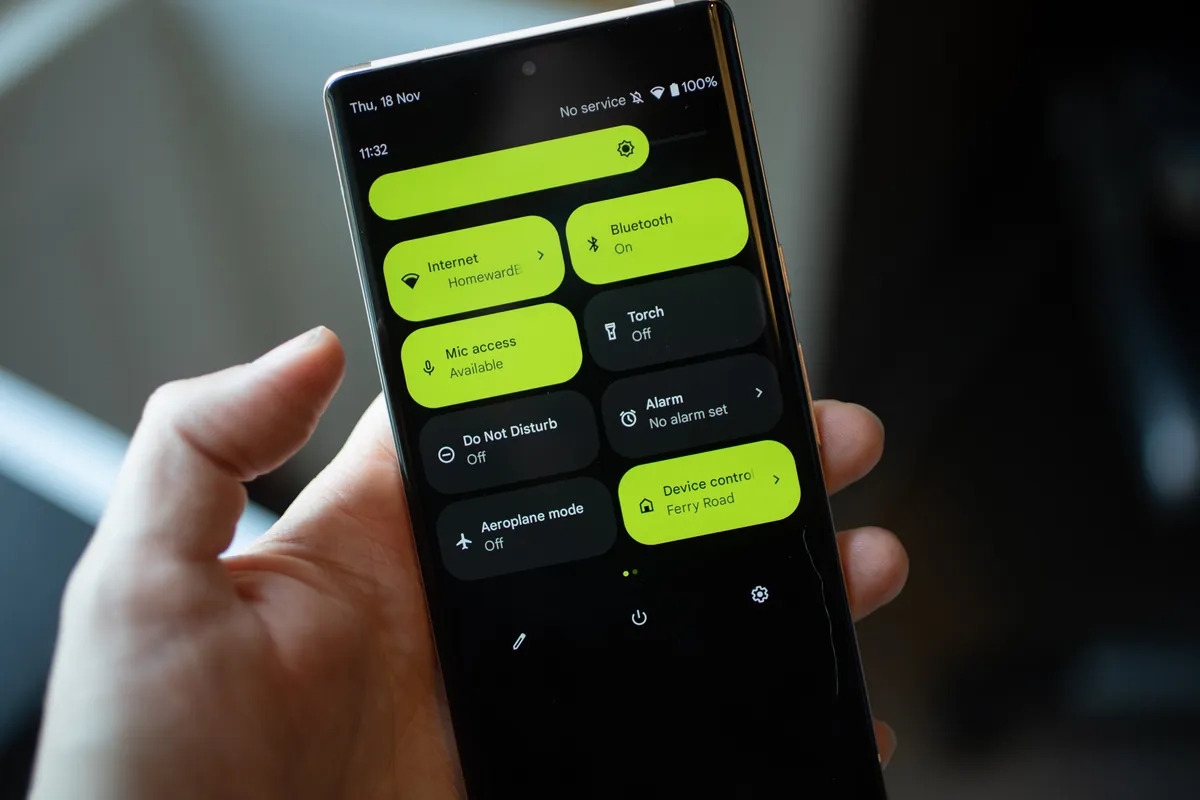
Introduction
Android menus are a crucial part of the User Interface (UI) in Android applications. They provide a consistent way for users to access various functions and settings. This guide covers the different types of menus available in Android, how to define and implement them, and best practices for creating user-friendly menus.
Types of Menus in Android
Android offers three primary types of menus: Options Menu, Context Menu, and Popup Menu. Each type serves a specific purpose and is used in different contexts within an application.
Options Menu
The Options Menu is the primary collection of menu items for an activity. It is where actions with a global impact on the app, such as "Search," "Compose email," and "Settings," are placed. This menu typically appears when the user presses the device MENU key.
Creating an Options Menu
To create an Options Menu, define it in an XML file and then inflate it in your activity's code.
-
Define the Menu in XML:
- Create a new XML file inside the
res/menu
directory of your project, for example,game_menu.xml
. - The XML file should contain the
<menu>
element as the root node, holding one or more<item>
elements. - Each
<item>
element represents a single menu item and can contain attributes likeandroid:id
,android:title
, andandroid:icon
.
xml
- Create a new XML file inside the
-
Inflate the Menu in Code:
- In your activity, override the
onCreateOptionsMenu()
method to inflate the menu resource. - Use the
MenuInflater
to inflate the menu resource into the providedMenu
object.
kotlin
override fun onCreateOptionsMenu(menu: Menu): Boolean {
val inflater: MenuInflater = menuInflater
inflater.inflate(R.menu.game_menu, menu)
return true
}java
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.game_menu, menu);
return true;
} - In your activity, override the
-
Handling Menu Item Selection:
- To handle the selection of menu items, override the
onOptionsItemSelected()
method. - In this method, check which menu item was selected using the
id
of the item.
kotlin
override fun onOptionsItemSelected(item: MenuItem): Boolean {
when (item.itemId) {
R.id.new_game -> {
// Handle new game selection
return true
}
R.id.quit -> {
// Handle quit selection
return true
}
else -> {
return super.onOptionsItemSelected(item)
}
}
}java
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.new_game:
// Handle new game selection
return true;
case R.id.quit:
// Handle quit selection
return true;
default:
return super.onOptionsItemSelected(item);
}
} - To handle the selection of menu items, override the
Context Menu
A Context Menu is a floating menu that appears when the user performs a touch-and-hold action on an element. It provides actions that affect the selected content or context frame. This menu is useful for elements requiring additional actions beyond the primary menu.
Creating a Context Menu
To create a Context Menu, override the onCreateContextMenu()
method in your activity or fragment.
-
Define the Context Menu in XML:
- While not mandatory, defining a Context Menu in an XML file is possible. However, creating it programmatically is more common.
-
Create Context Menu Programmatically:
- Override the
onCreateContextMenu()
method to create and populate the Context Menu. - Use the
ContextMenu
object provided by the system to add menu items.
kotlin
override fun onCreateContextMenu(contextMenu: ContextMenu, view: View, contextMenuInfo: ContextMenuInfo) {
super.onCreateContextMenu(contextMenu, view, contextMenuInfo)
val inflater = contextMenu.menuInflater
inflater.inflate(R.menu.context_menu, contextMenu)
}java
@Override
public void onCreateContextMenu(ContextMenu menu, View v, ContextMenuInfo menuInfo) {
super.onCreateContextMenu(menu, v, menuInfo);
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.context_menu, menu);
} - Override the
-
Handling Context Menu Item Selection:
- To handle the selection of Context Menu items, override the
onContextItemSelected()
method. - In this method, check which Context Menu item was selected using the
id
of the item.
kotlin
override fun onContextItemSelected(item: MenuItem): Boolean {
when (item.itemId) {
R.id.cut -> {
// Handle cut selection
return true
}
R.id.copy -> {
// Handle copy selection
return true
}
else -> {
return super.onContextItemSelected(item)
}
}
}java
@Override
public boolean onContextItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.cut:
// Handle cut selection
return true;
case R.id.copy:
// Handle copy selection
return true;
default:
return super.onContextItemSelected(item);
}
} - To handle the selection of Context Menu items, override the
Popup Menu
A Popup Menu displays a vertical list of items anchored to the view that invokes the menu. It is good for providing an overflow of actions related to specific content or options for the second part of a command. Actions in a Popup Menu do not directly affect the corresponding content; instead, they are used for extended actions.
Creating a Popup Menu
To create a Popup Menu, use the PopupMenu
class and its associated methods.
-
Create Popup Menu Programmatically:
- Use the
PopupMenu
constructor to create a new instance. - Set the anchor view using the
setAnchorView()
method. - Show the Popup Menu using the
show()
method.
kotlin
private fun showPopupMenu(view: View) {
val popupMenu = PopupMenu(context, view)
popupMenu.inflate(R.menu.popup_menu)
popupMenu.setOnMenuItemClickListener { item ->
when (item.itemId) {
R.id.share -> {
// Handle share selection
return@setOnMenuItemClickListener true
}
else -> {
false
}
}
}
popupMenu.show()
}java
private void showPopupMenu(View view) {
PopupMenu popupMenu = new PopupMenu(context, view);
popupMenu.inflate(R.menu.popup_menu);
popupMenu.setOnMenuItemClickListener(new PopupMenu.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem item) {
switch (item.getItemId()) {
case R.id.share:
// Handle share selection
return true;
default:
return false;
}
}
});
popupMenu.show();
} - Use the
Best Practices for Creating User-Friendly Menus
Creating user-friendly menus is crucial for providing a smooth and consistent experience throughout your application. Here are some best practices to keep in mind:
Use Clear and Concise Labels
Ensure that menu item labels are clear and concise. Avoid using abbreviations or jargon that might confuse users.
Use Icons Wisely
Icons can help users quickly identify menu items, but use them sparingly to avoid clutter. Ensure that icons are relevant to the action they represent.
Organize Menu Items Logically
Organize menu items logically so that related actions are grouped together. This makes it easier for users to find what they need quickly.
Provide Shortcut Keys
If your application supports devices with hardware keyboards, consider adding shortcut keys for menu items. This can enhance the user experience by allowing users to perform actions more efficiently.
Test Thoroughly
Thoroughly test your menus to ensure they are intuitive and easy to use. Test on different devices and screen sizes to ensure consistency across various platforms.
Dynamic Menu Items
Sometimes, adding menu items dynamically based on the user's context or device capabilities is necessary. Android provides several methods to achieve this:
Using addIntentOptions()
If uncertain that the user's device contains an application handling a specific Intent, use addIntentOptions()
to dynamically add menu items. This method searches for any applications that can perform the Intent and adds them to your menu.
kotlin
val menu = menuInflater.inflate(R.menu.game_menu, menu)
menu.addIntentOptions(
R.id.intent_share,
Intent.ACTION_SEND,
Intent.CATEGORY_DEFAULT,
null,
null,
Intent.FLAG_ACTIVITY_NEW_TASK or Intent.FLAG_ACTIVITY_CLEAR_TASK,
null,
null
)
java
Menu menu = menuInflater.inflate(R.menu.game_menu, menu);
menu.addIntentOptions(
R.id.intent_share,
Intent.ACTION_SEND,
Intent.CATEGORY_DEFAULT,
null,
null,
Intent.FLAG_ACTIVITY_NEW_TASK or Intent.FLAG_ACTIVITY_CLEAR_TASK,
null,
null
);
Final Thoughts
Android menus are a powerful tool for providing a consistent and intuitive user experience in your applications. By understanding the different types of menus available and following best practices for creating user-friendly menus, you can enhance the overall usability of your app. Whether creating an Options Menu, Context Menu, or Popup Menu, the key is to keep it simple, logical, and easy to use. With these guidelines, you can create menus that make your application stand out and provide a seamless experience for your users.