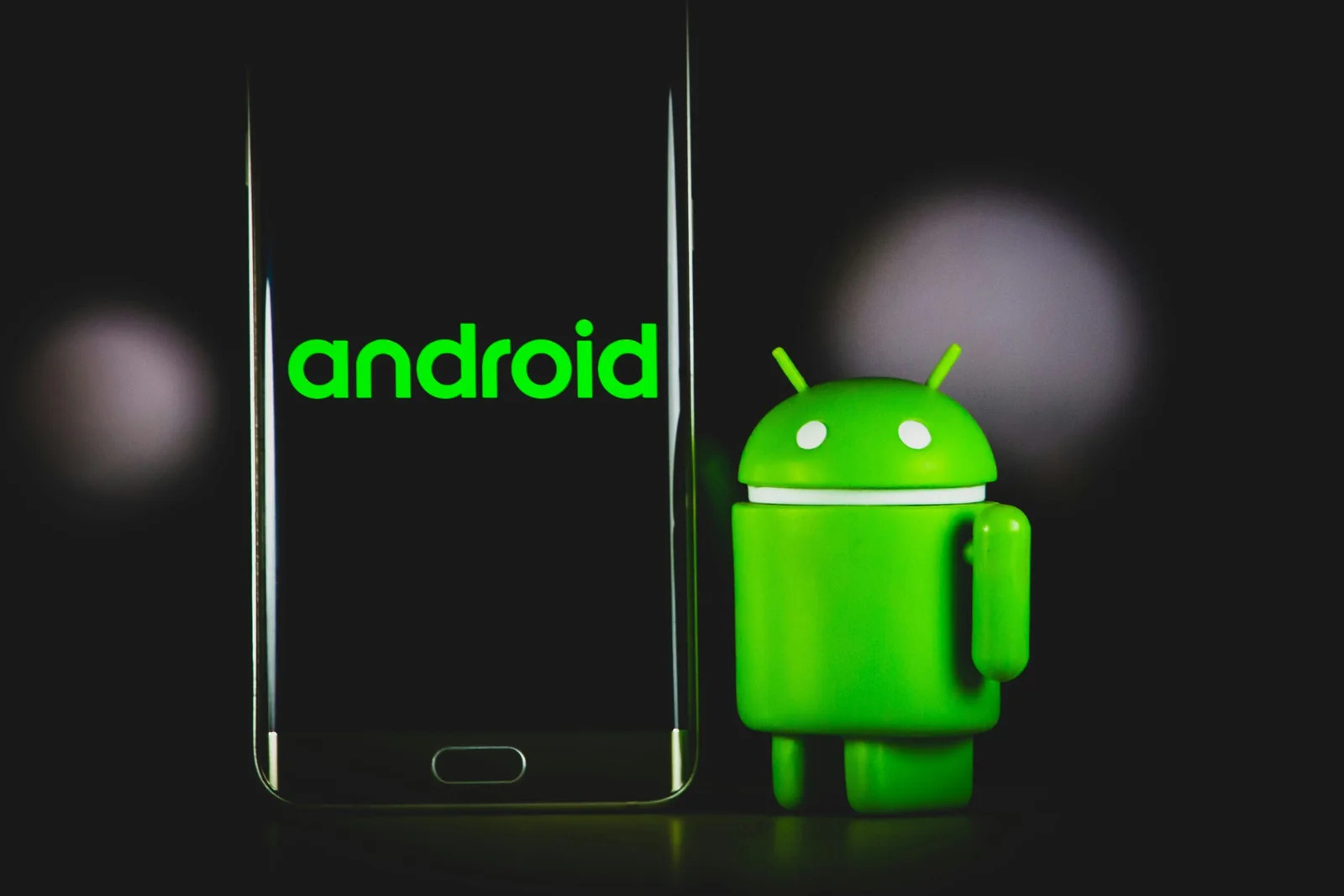
Introduction
Android app development involves creating software applications for devices powered by the Android operating system. With millions of devices running on Android, it has become the most popular operating system globally. This guide will walk you through the entire process of developing an Android app, from understanding the basics of Android architecture to designing, coding, testing, and launching your application.
What is Android App Development?
Android app development involves creating software applications that use the Android operating system as their platform. The process primarily focuses on coding, testing, and debugging applications that users can download and use on their devices. By learning how to develop Android apps, you can tap into a massive market of users and create innovative applications that can make a significant impact.
Basics of Android App Development
Understanding Android architecture is crucial for developers. The Android architecture has four main layers:
1. Linux Kernel
The Linux Kernel is the foundation of the Android operating system. It provides core system services like security, memory management, and process management. Think of the Linux Kernel as the engine of a car; just like how the engine powers the car, the Linux Kernel is the powerhouse of the Android operating system.
2. Libraries Layer
The Libraries layer contains pre-built libraries such as SQLite, OpenGL, and SSL. These libraries are like tools in a toolbox that developers can use to build their applications. SQLite is used for managing databases, OpenGL for graphics rendering, and SSL for secure network communication.
3. Runtime Layer
The runtime layer runs the codes of the application. It includes tools like Android Runtime (ART) and Dalvik Virtual Machine (DVM). The runtime layer is like the conductor in an orchestra; it brings all the different parts of an Android app together to create a functioning application.
4. Application Layer
The Application Layer is what the user sees on their devices. It is the face of the application. In other words, this is what users install and interact with. Understanding the Android architecture is essential for developers to build high-quality, scalable, and secure Android applications.
Android App Development Frameworks
Different development frameworks are available for Android app development. Some of the most popular frameworks include:
- React Native: A cross-platform framework that allows developers to build apps for both Android and iOS using JavaScript and React.
- Xamarin: A framework developed by Microsoft that allows developers to build cross-platform apps using C#.
- Flutter: An open-source framework developed by Google that allows developers to build natively compiled applications for mobile, web, and desktop from a single codebase.
The App Development Process
The app development process is a multi-step approach that involves planning, designing, coding, testing, and launching an application.
1. Planning and Developing the App Idea
Android app development is not a one-size-fits-all process, and there’s always room for customization. Therefore, planning the app idea before beginning is crucial.
Research
Research the market to see what apps have already been developed and how they’re doing. Determine their niche and target audience. The goal is to create an app that fills a need for consumers looking for the type of functionality you’re providing.
Ways to conduct market research:
- Read reviews of apps in the category you’re targeting to see what people say about them.
- Browse app stores (Google Play) and look at the categories to see what’s popular or has been trending in recent months.
- Read app reviews and look for common themes among them; see what people like and dislike about the app.
- Look at apps that are similar to your idea, see what they do differently from one another, and determine what your app will offer that they don’t.
- Look at the various ways apps in your category are marketed (e.g., promotion and advertising) to see what’s working well.
- Visit app stores, forums, or blogs within the exact niche for additional feedback.
- Use surveys to gather feedback and opinions from your target audience regarding the app idea.
Solve a Problem
Ask yourself what problem you’re trying to solve by developing an app for Android; plan features accordingly. In other words, generate an app idea based on the user’s needs. Examples of problems that an Android app can solve include:
- Organizing and editing photos.
- Purchasing products online from a mobile device.
- Finding the cheapest petrol station nearby.
- Planning and tracking exercise routines.
- Finding new ways to make a profit on the stock market.
The more specific you can be with the value your app provides to the user, the more likely they will download it.
2. Designing the App’s User Interface
Once you have a wireframe prepared and layout finalized, you can then use it to flesh out the rest of your app design. Your app’s design has a significant impact on usability and user experience. UI that is hard to use or unappealing can be a pain, causing your users to drop out and look elsewhere.
Fortunately, achieving this in Android can be straightforward, thanks to an abundance of resources. One of these is Google’s Material Design guideline. The Material Design guidelines contain tips, best practices, and methods for every UI component, from buttons to layouts. Following these tried-and-tested principles is the easiest way to achieve great UI with minimal guesswork.
Of course, there are some instances where you might want to deviate from the Android look and design your own from scratch. In these cases, having a proper app design process is paramount. You can check out our design primer here to get you started.
3. Developing the App’s Front-end and Back-end
With all the planning and pre-work ready, it’s time to put all the elements together. In this step, you’ll write the code for your Android app’s front-end and back-end components.
The front end is the part that the user sees—in this case, the app itself. It covers the user interface and the algorithms that control it. The back end, on the other hand, is where the main app functions and data are processed. This usually sits on a web server, receiving and sending instructions to the front end. The back end is connected to the front end via an API.
Unsurprisingly, this is the most critical part of development and where delays typically happen. That’s why thorough planning is crucial so that you can anticipate and prepare for any potential bottlenecks during coding. However, even the best plans will go awry if they are in the hands of incompetent developers. To avoid this, you’ll need to hire a talented team to work on your front-end and back-end code.
Experience plays a critical role in writing your code with minimal issues and delays.
4. Testing the App
Testing is an essential step in the app development process. It ensures that your app works as expected and is free from bugs. Here are some types of testing you should perform:
- Unit Testing: This involves testing individual components of your app to ensure they work correctly.
- Integration Testing: This involves testing how different components of your app work together.
- UI Testing: This involves testing the user interface to ensure it is intuitive and user-friendly.
- Performance Testing: This involves testing the performance of your app to ensure it runs smoothly on different devices.
- Security Testing: This involves testing the security of your app to ensure it is secure and protects user data.
5. Launching the App
Once you have tested your app thoroughly, it’s time to launch it. Here are some steps you should follow:
- Prepare for Launch: Make sure you have all the necessary resources ready, including marketing materials and a launch plan.
- Submit to App Stores: Submit your app to the Google Play Store or other app stores where you want it to be available.
- Promote Your App: Use various marketing strategies to promote your app and attract users.
Tools and Technologies Used in Android App Development
Integrated Development Environment (IDE)
The most popular IDE for Android app development is Android Studio. It provides a comprehensive set of tools for building, testing, and debugging Android apps.
Programming Languages
The primary programming language for Android app development is Kotlin, although Java is still supported. Other languages like JavaScript and XML are also used in conjunction with Kotlin or Java.
Development Frameworks
As mentioned earlier, different development frameworks are available for Android app development. Some of the most popular frameworks include React Native, Xamarin, and Flutter.
Libraries and APIs
Android provides a wide range of libraries and APIs that developers can use to build their applications. Some of the most commonly used libraries include SQLite for database management, OpenGL for graphics rendering, and SSL for secure network communication.
Official Android Documentation
Google offers comprehensive documentation on Android development through the Android Developer website. This documentation covers everything from getting started with Android Studio to building and testing your app. The official documentation includes tutorials, guides, and sample code to help developers get started with Android app development.
Tips and Valuable Resources
Here are some tips and valuable resources that can help you in your journey of developing an Android app:
- Start with a Plan: Always start with a clear plan and thorough research to ensure that your app idea is viable.
- Use Material Design: Use Google’s Material Design guidelines to create a user-friendly and visually appealing UI.
- Test Thoroughly: Test your app thoroughly to ensure it works as expected and is free from bugs.
- Hire a Talented Team: Hire a talented team to work on your front-end and back-end code to avoid delays and issues.
- Follow Best Practices: Follow best practices for coding, testing, and debugging to ensure that your app is high-quality and scalable.
By following the steps outlined in this guide, you can create a high-quality Android app that meets the needs of your target audience. Use the right tools and technologies, follow best practices, and test your app thoroughly to ensure it is successful. With the right approach and resources, you can turn your app idea into a reality and make it available to millions of users worldwide.