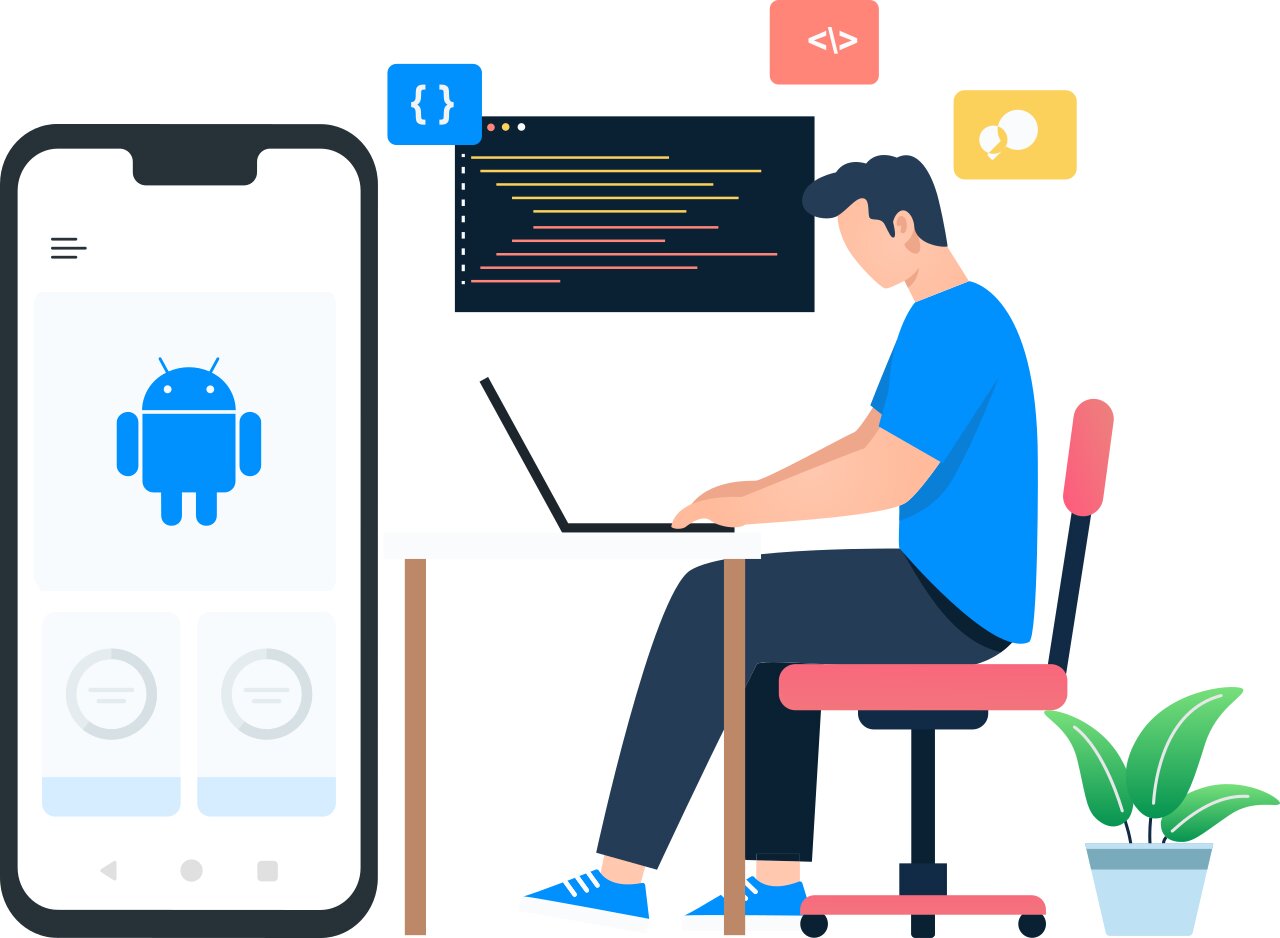
Getting Started with Android App Development
Creating an Android app can seem challenging, especially for beginners. However, with the right tools, tutorials, and tips, anyone can develop a functional and user-friendly app. This guide will cover essential steps, tools, and resources needed to build a successful mobile application.
Understanding the Basics
- Programming Languages: Android app development primarily uses Java or Kotlin. Java is more conventional and has a larger community, while Kotlin is preferred for its modern syntax and interoperability with Java.
- Object-Oriented Programming (OOP): Familiarity with OOP concepts such as classes, objects, inheritance, polymorphism, and encapsulation is essential.
- XML Basics: Understanding XML is vital for designing app layouts. XML (Extensible Markup Language) defines the structure and layout of your app's user interface.
Setting Up Your Development Environment
- Installing Android Studio: Android Studio is the official Integrated Development Environment (IDE) for Android app development. It provides a comprehensive set of tools for building, testing, and debugging your app.
- System Requirements: Ensure your computer meets the system requirements for running Android Studio. A quad-core CPU, 16GB RAM, and an NVMe SSD are recommended for smooth performance.
- Downloading and Installing: Follow the instructions provided by Google to download and install Android Studio. Detailed setup instructions can be found on the official Android Developers website.
Creating a New Project
Once the development environment is set up, it's time to create a new project in Android Studio.
Step-by-Step Guide to Creating a New Project
- Launch Android Studio: Double-click the Android Studio icon to launch the application.
- Welcome to Android Studio Dialog: In the welcome dialog, click on "New Project."
- New Project Window: The New Project window will open with a list of templates provided by Android Studio. Select the "Empty Activity" template, which is ideal for beginners and provides a simple project structure.
- Configure Your Project:
- Name: Enter the name of your project.
- Package Name: Leave the package name as is. This will organize your files in the file structure.
- Save Location: Leave the save location as is. Take note of where the project files will be stored.
- Minimum SDK: Select an API level that suits the range of devices you wish your app to support. For example, selecting API 24: Android 7.0 (Nougat) ensures your app can run on devices with this minimum version or higher.
Adding UI Elements and Functionality
After setting up your project, it's time to add user interface elements and functionality.
Understanding UI Elements
- View and ViewGroup: The structure of a user interface for an Android application consists of a hierarchy of View and ViewGroup objects. ViewGroup acts as containers that establish the structural layout design.
- Relative Units: To maintain functionality on different screen sizes across devices, it's essential to specify view dimensions using relative units such as density-independent pixels (dp).
Adding Interactivity
- Click Listeners: To allow interaction within views, you can attach click listeners which define behaviors when items like buttons are activated by a user’s touch.
- Java or Kotlin Code: Employ Java or Kotlin to incorporate fundamental functionalities. For example, creating an app experience that engages and accommodates users seamlessly involves defining behaviors in code.
Mastering Android Components
Android app development involves mastering various components to create a comprehensive and user-friendly app.
Activities
- Understanding Activities: An Activity is a single screen in your app that can perform specific tasks. Learn how to create, open, and navigate between activities. Understand the lifecycle methods of activities and how they are invoked.
- Activity Lifecycle Methods: Topically, what kind of code you should have in each lifecycle method is crucial. For example, the
onCreate()
method is called when the activity is created, while theonDestroy()
method is called when the activity is destroyed.
Fragments
- Understanding Fragments: A Fragment is a piece of an activity that can be used independently. Learn why you would use a Fragment instead of an Activity or together with an Activity. Understand the lifecycle methods of Fragments and how they are related to lifecycle methods of Activities.
- Fragment Lifecycle Methods: Similar to Activities, Fragments also have lifecycle methods like
onCreate()
,onResume()
, andonPause()
. These methods help manage the state of the Fragment.
Choosing the Right Development Stack
Choosing the right development stack is crucial for building a robust and scalable app.
Architectures
- MVC, MVP, MVVM: Learn about architectures like Model-View-Controller (MVC), Model-View-Presenter (MVP), and Model-View-ViewModel (MVVM). These architectures help structure your code and make it easier to manage.
- Understanding Each Architecture: Read articles and try implementing each architecture to understand their strengths and weaknesses. For example, MVVM is preferred for its simplicity and ease of use with data binding.
Building a User Interface with Jetpack Compose
Jetpack Compose is a modern UI toolkit for building native Android UIs. It allows you to write UI code in Kotlin, providing a declarative and compositional way of building user interfaces.
Getting Started with Jetpack Compose
- Prerequisites: Basic Kotlin knowledge is required to start with Jetpack Compose.
- Creating a Project with Jetpack Compose: Use the Empty Activity template provided by Android Studio to create a project. This template includes starter code to get you started with Jetpack Compose.
- Customizing Your App: Use Kotlin and Jetpack Compose to customize your app. For example, you can create an app that lets users customize their introduction by updating text and UI elements.
Testing and Debugging Your App
Testing and debugging are critical steps in ensuring your app is stable and functional.
Unit Testing
- Writing Unit Tests: Use JUnit to write unit tests for your code. This helps catch bugs early in the development process and ensures that individual components of your app are working correctly.
- Mocking Dependencies: Use mocking libraries like Mockito to isolate dependencies and make your tests more reliable.
UI Testing
- Using Espresso: Use Espresso for UI testing. This framework allows you to write tests that interact with your app's UI, ensuring that it behaves as expected.
- Automating Tests: Automate your tests using tools like Gradle. This helps streamline the testing process and ensures that your app is thoroughly tested before release.
Publishing Your App
Once you have developed and tested your app, it's time to publish it on the Google Play Store.
Preparing Your App for Launch
- Optimizing Performance: Optimize your app's performance by reducing its size and improving its loading time. Use tools like Proguard and R8 to reduce APK size.
- Following Guidelines: Follow the guidelines set by Google for publishing apps on the Play Store. Ensure that your app meets all the requirements and complies with the store's policies.
Submitting Your App
- Creating a Developer Account: Create a developer account on the Google Play Console if you haven't already.
- Uploading Your App: Upload your app to the Play Store, providing all necessary details such as screenshots, descriptions, and pricing information.
- Review Process: Go through the review process, which may take several days or weeks depending on the complexity of your app.
Additional Resources
For those looking to dive deeper into Android app development, here are some additional resources:
- Android Developers Official Website: The official website of Android Developers provides comprehensive guides, tutorials, and documentation for all aspects of Android app development.
- GeeksforGeeks Android Tutorial: GeeksforGeeks offers an extensive Android tutorial covering both basic and advanced concepts. This resource is ideal for both beginners and experienced developers.
- Reddit Community: The r/androidapps community on Reddit is a great place to ask questions, share knowledge, and learn from other developers.
- YouTube Tutorials: YouTube channels like Rahul Pandey's Android tutorials offer step-by-step guides on building specific types of apps, such as a tip calculator.
By leveraging these resources and following the tips outlined in this article, you can embark on your journey in Android app development with confidence.