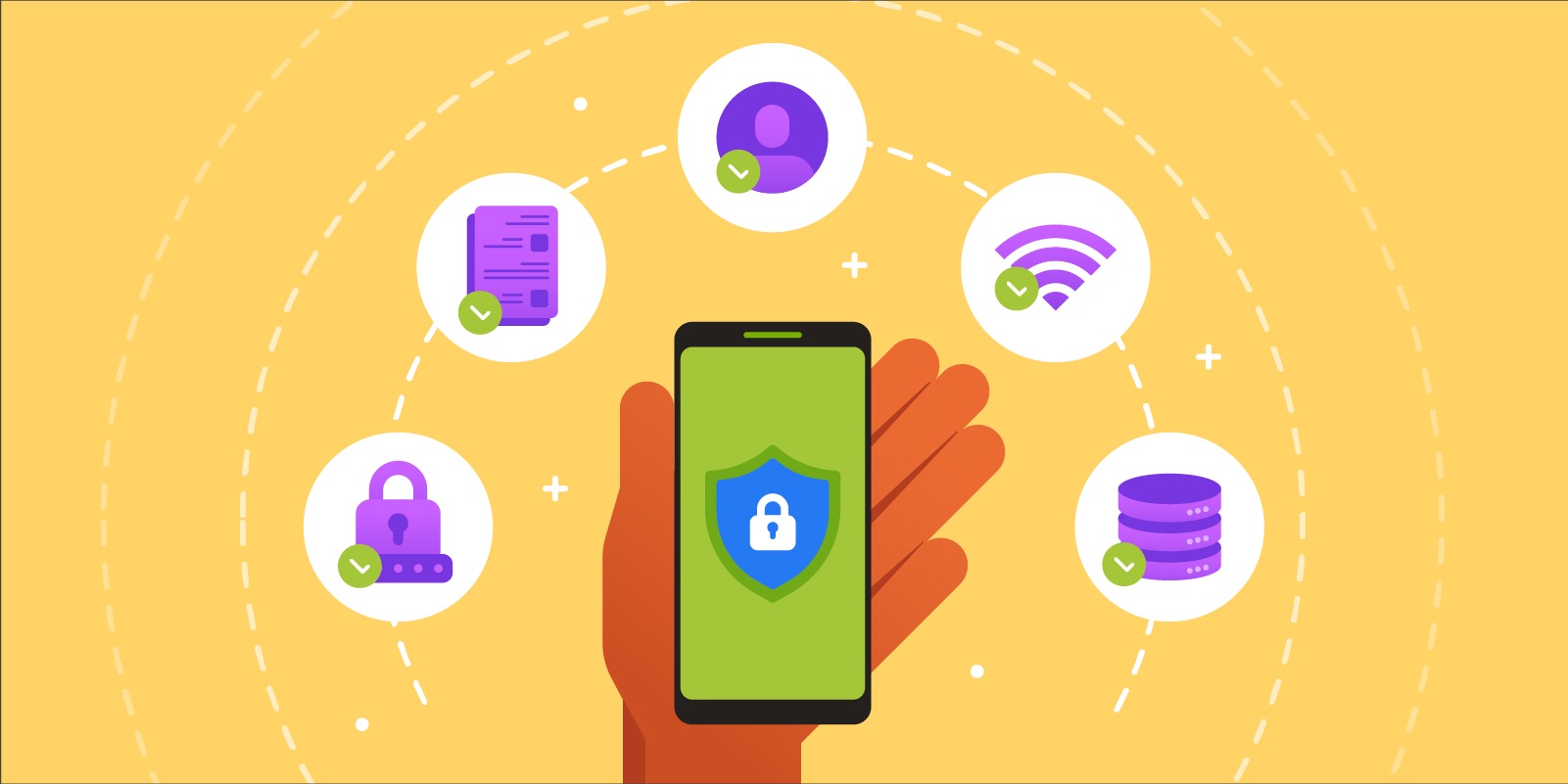
Understanding Android Security Challenges
Android's open nature and the vast ecosystem of devices make it a challenging platform to secure. Here are some key challenges:
-
Fragmentation: The Android ecosystem is highly fragmented, with numerous devices and system versions. This fragmentation makes it difficult to ensure that all devices are updated with the latest security patches, leaving some devices vulnerable to known exploits.
-
Decompilation: Android apps can be decompiled, which means that an attacker can reverse-engineer your code and potentially modify it. This is a significant risk, especially if your app contains sensitive data or functionality.
-
Malware and Threats: Android is a popular target for malware and other types of threats. These can range from simple adware to sophisticated banking trojans.
-
Data Protection: Ensuring the protection of user data is crucial. This includes not only sensitive information like passwords but also personal data such as location and contact details.
Build Integrity Verification
One of the most effective ways to enhance Android security is through build integrity verification. This involves ensuring that the code and resources used in your app have not been tampered with during the build process.
Advantages of Using Build Integrity Verification
-
Protection Against Tampering: Build integrity verification makes it difficult for attackers to modify your application's code without being detected. This includes protection against tools like Lucky Patcher, which are commonly used to bypass in-app purchase mechanisms.
-
Ease of Implementation: Implementing build integrity verification is relatively straightforward and can be integrated into your continuous integration (CI) pipeline.
-
Commonality: Since this method is not commonly used, it provides an additional layer of security that many attackers may overlook.
How to Implement Build Integrity Verification
To implement build integrity verification, you can follow these steps:
-
Calculate Checksum:
- Retrieve the
classes.dex
file from your APK. - Calculate the checksum for this file.
- Retrieve the
-
Store Checksum:
- Store the calculated checksum in a secure location, such as
strings.xml
.
- Store the calculated checksum in a secure location, such as
-
Verify Checksum:
- During the build process, verify that the stored checksum matches the newly calculated one.
- If there is a mismatch, throw an exception indicating that the build is invalid.
Here’s an example of how you can automate this process using Gradle tasks:
gradle
static def getChecksum() {
def command = "crc32 PATH_TO_CLASSES_DEX"
return command.execute().text
}
task verifyChecksum() {
doLast {
if (getChecksum() != getCachedChecksum()) {
throw new GradleException("Invalid checksum")
}
}
}
task updateChecksum() {
doLast {
// Store currentChecksum in strings.xml
// Implementation details left out for brevity
}
}
// Example bash script for CI system
set -e
./gradlew clean
./gradlew app:assembleRelease
set +e
if ./gradlew verifyChecksum; then
echo "Checksum valid"
else
set -e
./gradlew updateChecksum
./gradlew clean
./gradlew app:assembleRelease
./gradlew verifyChecksum
fi
This script ensures that before each build, the clean
task is executed to remove any previously calculated checksums. The verifyChecksum
task checks if the newly calculated checksum matches the stored one. If there is a mismatch, it updates the stored checksum and rebuilds the APK.
Certificate Pinning
Certificate pinning is another crucial security measure that involves pinning the expected SSL/TLS certificate to ensure that only trusted certificates are accepted by your app.
Why Use Certificate Pinning?
-
Prevent Man-in-the-Middle Attacks: By pinning the expected certificate, you prevent man-in-the-middle attacks where an attacker intercepts communication between your app and the server.
-
Enhance Trust: Certificate pinning enhances trust by ensuring that only the expected certificate is accepted, reducing the risk of impersonation attacks.
How to Implement Certificate Pinning
-
Obtain Trusted Certificate: Obtain the trusted SSL/TLS certificate from your server.
-
Add Certificate to App: Add the trusted certificate to your app's resources.
-
Verify Certificate: During HTTPS connections, verify that the received certificate matches the pinned one.
Here’s an example of how you can implement certificate pinning in an Android app:
java
import java.security.KeyStore;
import java.security.SSLContext;
import javax.net.ssl.SSLContext;
import javax.net.ssl.TrustManager;
import javax.net.ssl.TrustManagerFactory;
import javax.net.ssl.X509TrustManager;
public class CertificatePinning {
public static void main(String[] args) throws Exception {
// Load trusted certificate from resources
InputStream in = CertificatePinning.class.getResourceAsStream("/trusted_cert.crt");
KeyStore trustStore = KeyStore.getInstance("BKS");
trustStore.load(in, "password".toCharArray());
// Create an instance of TrustManagerFactory
TrustManagerFactory trustManagerFactory = TrustManagerFactory.getInstance(TrustManagerFactory.getDefaultAlgorithm());
trustManagerFactory.init(trustStore);
// Get an instance of X509TrustManager
X509TrustManager trustManager = (X509TrustManager) trustManagerFactory.getTrustManagers();
// Create an SSLContext with the custom trust manager
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(null, new TrustManager[]{trustManager}, null);
// Use the SSLContext for HTTPS connections
// Example: https://example.com
URL url = new URL("https://example.com");
HttpsURLConnection connection = (HttpsURLConnection) url.openConnection();
connection.setSSLSocketFactory(sslContext.getSocketFactory());
}
}
License Verification
License verification is another important security measure that ensures your app is running with a valid license.
Why Use License Verification?
-
Prevent Piracy: License verification prevents piracy by ensuring that only licensed copies of your app are running.
-
Enhance Revenue: By ensuring that users are running licensed copies, you can enhance revenue streams through proper licensing mechanisms.
How to Implement License Verification
-
Generate License Key: Generate a unique license key for each user.
-
Store License Key: Store the license key securely in your app's database or preferences.
-
Verify License Key: During app startup, verify that the stored license key matches the expected one.
Here’s an example of how you can implement license verification in an Android app:
java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class LicenseVerification {
public static boolean verifyLicense(String storedLicenseKey, String expectedLicenseKey) {
return storedLicenseKey.equals(expectedLicenseKey);
}
public static void main(String[] args) throws IOException {
// Example: storedLicenseKey and expectedLicenseKey are retrieved from database or preferences
String storedLicenseKey = "stored_license_key";
String expectedLicenseKey = "expected_license_key";
if (!verifyLicense(storedLicenseKey, expectedLicenseKey)) {
// Handle invalid license key
System.out.println("Invalid license key");
return;
}
// App is running with a valid license
System.out.println("App is running with a valid license");
}
}
Final Thoughts
Enhancing Android security is a multifaceted task that requires a combination of various security measures. Build integrity verification, certificate pinning, and license verification are three critical techniques that can significantly improve the security of your Android app. By implementing these measures, you can protect your app from various types of attacks and ensure that your users have a secure experience.
Related Articles
-
Android App Development: How to Build a Great Mobile Product
- This article provides insights into the process of building a successful Android app, including considerations for system versions and multiple devices.
-
Android Animation Libraries You Should Know
- This article discusses the importance of in-app animations and highlights some of the great animation libraries used in Android projects.
By following these guidelines and integrating these security measures into your development process, you can create a more secure and trustworthy Android application.