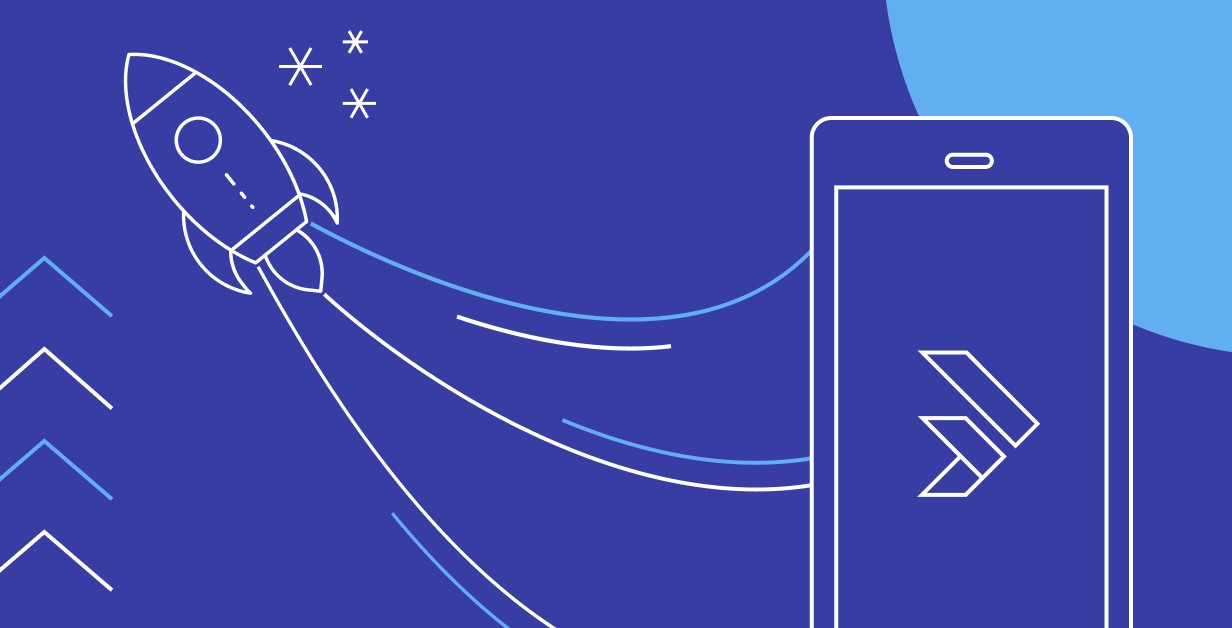
Introduction to Flutter
Flutter, a cross-platform development toolkit by Google, enables developers to build natively compiled applications for mobile, web, and desktop from a single codebase. This framework uses the Dart programming language, offering a rich set of pre-designed widgets for creating beautiful, responsive UIs. One standout feature is hot reload, allowing developers to see changes in real-time, significantly speeding up development.
Key Features of Flutter
- Cross-Platform Development: Build apps for multiple platforms using a single codebase, reducing time and cost.
- Widget-Based UI: Utilize a library of pre-designed elements to create complex UIs easily.
- Hot Reload: See changes in real-time without restarting the app, useful for rapid prototyping and debugging.
- Dart Language: Dart's clean and concise syntax makes it ideal for rapid development.
- Integrated Tools: Tools like Flutter DevTools help with debugging, testing, and performance optimization.
Setting Up the Flutter Environment
1. Check System Requirements
Ensure your computer meets the minimum requirements for Flutter, including the latest version of Android Studio and the Java Development Kit (JDK). Check the official Flutter website for detailed requirements.
2. Download Flutter SDK
Visit the official Flutter website to download the SDK. Extract the files to a preferred location. The extracted folder contains the Flutter bin directory, which needs to be added to your system's PATH.
3. Update Path
Add the Flutter bin directory to your system's PATH:
- Windows: Right-click on "Computer" or "This PC," select "Properties," then "Advanced system settings," and click "Environment Variables." Under "System Variables," find the "Path" variable, click "Edit," then "New," and enter the path to the Flutter bin directory.
- macOS: Open Terminal and run
export PATH=$PATH:/path/to/flutter/bin
. - Linux: Open Terminal and run
export PATH=$PATH:/path/to/flutter/bin
.
4. Install Android Studio
If Android Studio isn't installed, download it from the official website. Open it and install the Flutter and Dart plugins from the plugin marketplace.
5. Set Up Emulator
Create an Android Virtual Device (AVD) in Android Studio to test apps without a physical device:
- Open Android Studio.
- Go to
File
>Settings
. - Navigate to
System Settings
>Android SDK
. - Click on
Virtual Devices
and thenCreate Virtual Device
. - Choose a device and click
Next
. - Select an emulator image and click
Next
. - Configure the emulator settings as needed and click
Finish
.
6. Run Flutter Doctor
Open a terminal and type flutter doctor
. This command checks your environment and displays a report on the status of your installation. Follow any additional steps it suggests.
7. Create a New Project
Start a new project using flutter create my_app
. Navigate into the project directory with cd my_app
.
8. Run Your App
Connect a physical device or start the emulator, then use flutter run
to launch your app.
Debugging with Flutter DevTools
Flutter DevTools is a suite of tools for inspecting your app, debugging issues, and improving performance.
- Open DevTools: Run
flutter pub global activate flutter_devtools
in your terminal. - Launch DevTools: Open a browser window and navigate to
http://127.0.0.1:8080/
. - Performance Insights: Use the Performance tab for insights into your app's performance.
- Memory Profiler: Use the Memory tab to profile memory usage.
- Dart Profiler: Use the Dart tab to profile Dart code execution.
Preparing a Web App for Release
1. Compile the App
Compile the app using flutter build web
. This generates necessary files in the build/web
directory, including HTML, CSS, and JavaScript.
2. Choose a Web Hosting Service
Select a web hosting service to deploy your app. Popular options include GitHub Pages, Firebase Hosting, and Netlify. Generally, upload the contents of the build/web
directory to the hosting service.
3. Decide on a Web Renderer
Choose a web renderer for your app. Flutter supports HTML and CanvasKit renderers. The HTML renderer offers good performance for most apps, while CanvasKit is better for advanced graphics or animations.
Tips for Effective Use of Flutter
Organize Your Project Structure
Keep your project structure organized by placing files in logical folders like lib
, assets
, and test
. Use meaningful names for classes and methods.
Utilize Widgets Efficiently
Choose between stateless and stateful widgets based on content type. Stateless widgets suit static content, while stateful widgets are better for dynamic content.
Hot Reload is Your Friend
Maximize the hot reload feature to see changes instantly without restarting the app, useful during rapid prototyping and debugging.
Testing is Crucial
Ensure app quality by writing unit tests for logic and widget tests for UI. Use tools like the test
package in Dart for writing tests.
Version Control with Git
Use version control with Git, committing changes frequently and using branches for features. This helps track changes and collaborate with other developers.
Optimize Performance
Avoid rebuilding entire widgets to optimize performance. Use keys to preserve state and improve rendering efficiency.
Documentation Matters
Comment your code and use README files for documentation. This aids in understanding the codebase for other developers and future maintenance.
Stay Updated
Keep up with the latest releases of Flutter and its dependencies. Use commands like flutter upgrade
to get the latest version.
Community is a Resource
Join forums and attend meetups to connect with other developers. Ask questions and share knowledge to improve your skills.
Plugins Can Save Time
Find useful packages on pub.dev to save time during development. Plugins can assist in tasks like debugging, testing, and performance optimization.
Final Thoughts
Mastering Flutter installation is achievable by following these steps carefully. Start by checking system requirements, downloading the SDK, and updating the PATH. Install Android Studio and set up an emulator to test your apps. Use Flutter DevTools for debugging and performance insights. Prepare your web app for release by compiling it and choosing a web hosting service. Stay updated with the latest releases and utilize community resources to improve your skills.
Technology continues to shape our world at an incredible pace. Whether diving into Flutter for app development, exploring new gadgets, or keeping tabs on the latest trends, understanding these tools can open up endless possibilities. Stay curious and never stop learning. In this dynamic world of bytes and circuits, the next big thing is always just around the corner.